14 Jan 2025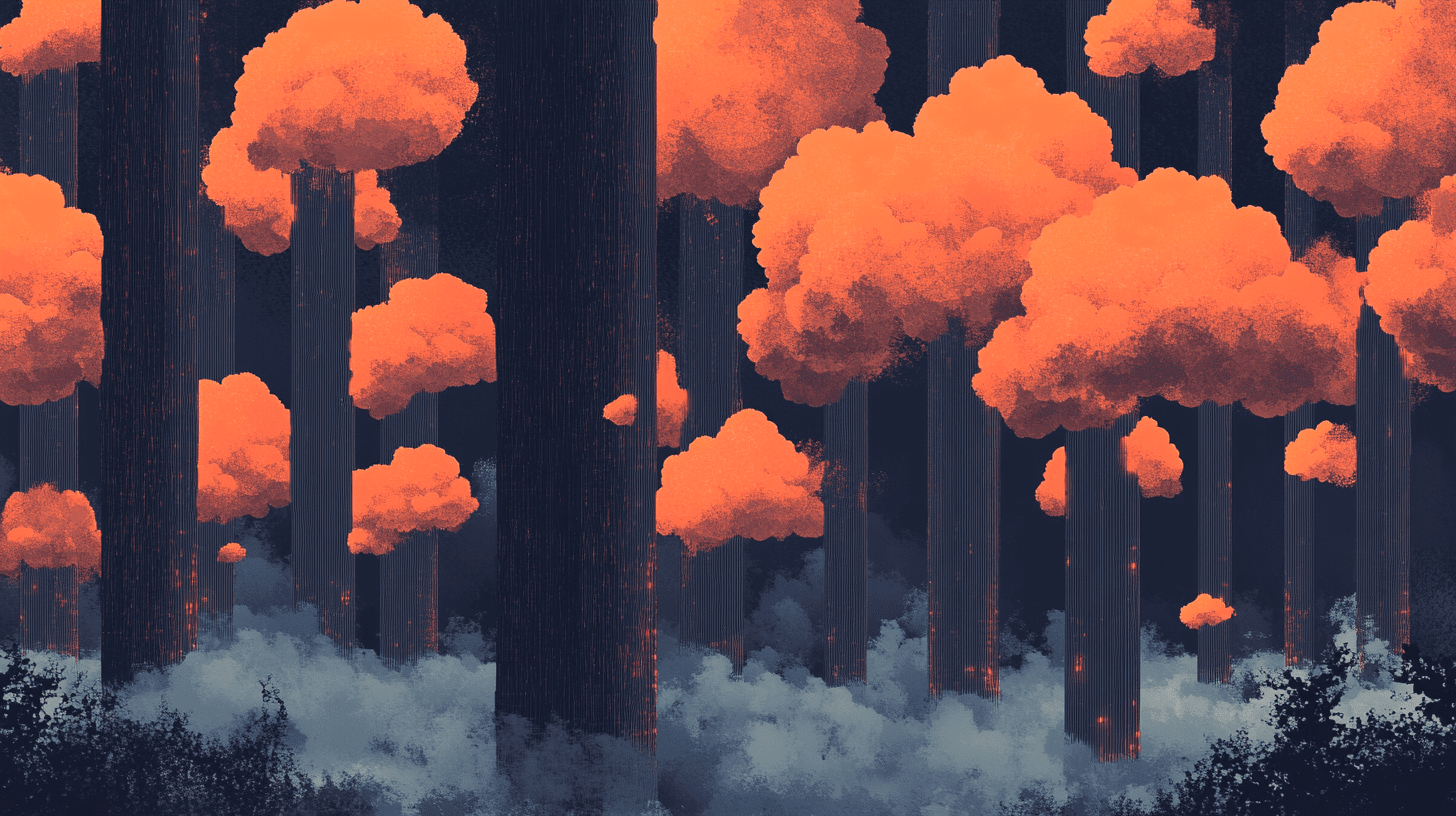
I had to work with Cloudflare Workers recently, and everything worked well until one day one of the HTTP calls I was doing started to fail.
When I ran the same piece of code locally it worked (obviously!). But pushed and ran through Cloudflare Workers, it failed. This was the first step in what then became a day-long trip into the rabbit hole of debugging. After a couple of hours of debugging "live" (by pushing my code, hitting the server, and checking logs), I finally discovered that my issue was that the HTTP endpoint I targeted had a rate limit, based on the originating IP. And when doing calls from Cloudflare, sharing the same IP with other workers, the IP already had hit the limit and my calls would fail.
That made me start digging into better ways to locally test CFW production code, and I discovered the wrangler dev
mode. At first I thought this would spin my code on a real remote server and broadcast the console.log
locally to my terminal, but no, it's a minimal version of CFW that runs locally. Not exactly the same as a staging env, but pretty close.
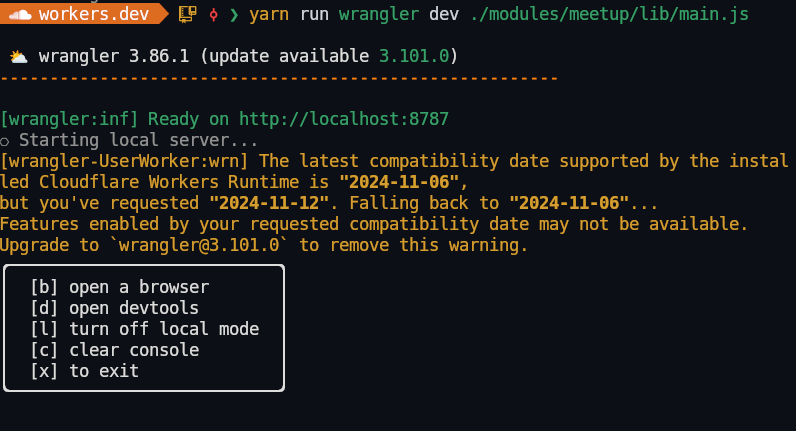
The main difference with running my scripts locally through unit tests is that when using wrangler dev
, my code is bundled with esbuild
and the bundled version is executed. This opened a whole new category of problems and questions to me.
First, I realized that the bundle size was way too big for what my function was actually doing. I had ~100 lines of code at most, but my bundle was several megabytes of minified code. Surely, something wasn't right. By inspecting the bundled code I realized that it had bundled all my dependencies and subdependencies.
But, isn't it supposed to do tree shaking?
I had read that esbuild
was the new hotness, and that it should do tree shaking my dependencies automatically, keeping only what I would actually use. But somehow, it didn't seem to work.
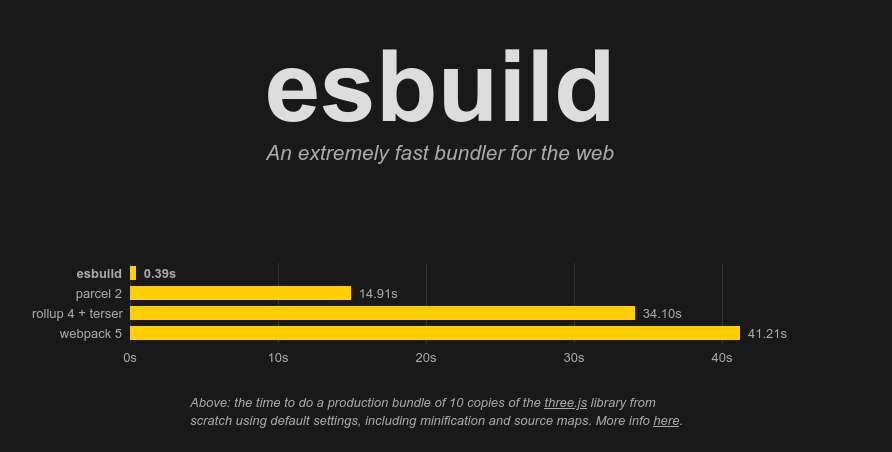
What I learned is that tree-shaking is not possible through the virtue of esbuild
alone. Modules have to be in ESM as well (so, basically using import
rather than require
) for it to actually work. So I updated my dependencies to their latest versions; most of them are now ESM-compliant. I managed to upgrade all my deps to ESM, and with that, esbuild
was now able to tree shake the final bundle, reducing my filesize footprint to something 10 times smaller \o/.
ESMify all the things
One of the dependencies was actually one of my own modules, firost, and let me tell you that converting a CommonJS module to ESM is not a trivial task. It's certainly doable, but it does take some time, especially when you have several intertwined modules, some in CommonJS and others in ESM.
I especially had to be careful to use named exports rather than God Objects in my files, to avoid pulling all dependencies with a greedy import. The restructuring of files and import was tedious and long. I also had to ditch Jest (that does not support ESM) in favor of Vitest. I also updated ESLint to its latest version, which finally also supports ESM!
Lodash, you're next
The only dependency I didn't manage to shave off was lodash
. I really like lodash, especially the _.chain().value()
syntax, which I think makes expressing complex pipelines easier. But lodash
still seems to be loaded as a monolithic block, even though I'm only using a few of its methods. I didn't dig too much into how to load it in a more clever way, but that's on my TODO list.
I also needed to include cheerio
(because my worker is doing some scraping + HTML extraction), but couldn't find a way to load a leaner alternative (domjs
is roughly the same size, and I prefer the API from cheerio
)
08 Jan 2025Recently, I found myself connecting to remote machines quite often. I have to debug remote servers for work or connect through ssh
to an emulation handheld console I just bought (more posts coming on that later).
But I've configured my local zsh
so much that when I connect to a bare remote server I feel a bit lost. No colors to differentiate folders and files. No tabbing through completion. Even simple things like backspace or delete key do not always work.
So this time, I built a very minimal .zshrc
that I intend to scp
to a remote server whenever I need to do some work there, just to make my life easier. I tried it on the aforementioned console, and it helped a lot, so I'm gonna share it here.
Fix the keyboard
export TERM=xterm-256color
bindkey "^[[3~" delete-char # Delete
bindkey "^?" backward-delete-char # Backspace
bindkey "^[OH" beginning-of-line # Start of line
bindkey "^[OF" end-of-line # End of line
Starting with the basics, I ensure my terminal type is set to xterm-256color. It should fix most keyboard issues. But just to be sure I actually did define the keycodes for delete, backspace as well as start and end of line.
The ^[
and ^?
chars here are not real characters, but escape characters. In vim, you have to press Ctrl-V
, followed by the actual key (so, backspace, or delete, etc) to input it correctly. I found that various servers had this mapped differently, so you might have to manually change it if it doesn't work for you.
Completion
autoload -Uz compinit
compinit
zstyle ':completion:*' menu select
This will enable a much better completion than the default one. Now, whenever there are several possible solutions when pressing tab
, the list of possibilities will be displayed, and you can tab
through them as they are getting highlighted.
Colors
autoload -U colors && colors
export COLOR_GREEN=2
export COLOR_PURPLE=21
export COLOR_BLUE=94
export LS_COLORS="di=38;5;${COLOR_GREEN}:ow=38;5;${COLOR_GREEN}:ex=4;38;5;${COLOR_PURPLE}:ln=34;4;${COLOR_BLUE}"
zstyle ':completion:*' list-colors ${(s.:.)LS_COLORS}
I then added a bit of color. I defined a few variables to better reference the colors. Those are mapped based on the color palette I'm using in my local kitty
. They would probably be different on your machine, so you should also adapt it.
The LS_COLORS
definition sets the directories in green, executable files in purple and symlinks in blue. This simple change already makes everything much easier to grok. The zstyle
line also applies those colors to the tab completions \o/.
Aliases
alias v='vi'
alias ls='ls -lhN --color=auto'
alias la='ls -lahN --color=auto'
alias ..='cd ..'
function f() {
find . -name "*$1*" | sed 's|^./||'
}
I added some very minimal aliases; those that are embedded in my muscle memory. I have much more locally, but I went for the minimal amount of aliases to make me feel at home. I also didn't want to have to install any third party (even if exa
, fd
and bat
would sure would have been nice).
v
is twice as fast to type as vi
. Some better ls
and la
(for hidden files). A quick way to move back one level in the tree structure, and a short alias to find files based on a pattern. Those are simple, but very effective.
Prompt
PS1="[%m] %{[38;5;${COLOR_GREEN}m%}%~/%{[00m%} "
And finally a left-side prompt to give more information of where I am. It starts with the name of the current machine, so I can easily spot if I'm on a remote session or locally, then the current directory (in green, once again, as is my rule for directories).
The wrapping %{
and %}
are needed around color espace sequences, to tell zsh
that what's inside doesn't take any space on the screen. If you omit them, you'll see that what you type is offset on the right by a large amount.
I actually like to replace the %m
with a machine-specific prefix, to more easily see where I am.

Here, for example, you can see I'm connected to my handheld console (I added the SNES-like colored button), currently in the /roms2/
directory and I'm tabbing through completions in the ./n64/games/
folder.
And that's it. A very minimal .zshrc
for when I need to get my bearings on a new remote server and still be able to do what I want quickly.
06 Jan 2025I've been working on my Airtable and Make automations, and I wanted to share a small trick I've implemented to improve error handling and visibility.
I use Airtable interfaces equipped with various buttons that, when clicked, trigger webhooks in Make, that in turn update my Airtable record with additional data. However, I found it quite frustrating that there was no visual feedback indicating the status of the webhook. Was it still running? Did it succeed? Did it fail? I had to open my Make dashboard each time, which was far from ideal.
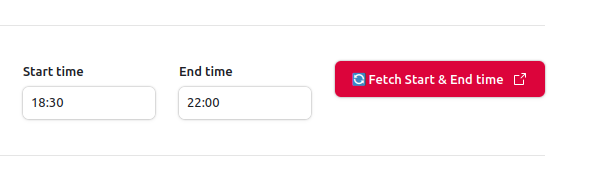
So I added a simple status feature. I added a new field called automationStatus
to my table. It's a select with three states: OK
(default), In Progress
, or Error
. By default, it's set to OK.
Now, when the webhook starts, instead of fetching the relevant Airtable record, it updates it instead by changing its automationStatus
to In Progress
. The Make module to update an Airtable record also returns the whole record, so I don't need to actually fetch it. I display the status next to the button, so now I can get visual feedback.

At the end of a successful scenario, when I update the record with new data, I also set the automationStatus
back to OK
. And if any module fails along the way I add an Error Handler to set the automationStatus
to Error
.
For some very nasty scenarios, I even went further and added the automationScenarioUrl
and automationErrorDetails
, so I could have more visibility on what was really happening, and quickly click on the link to get to the Make Scenario page.
This approach of course has limitations (there is no history, and all automations of a given record share the same field), but it is already way better than what I used to have (ie. nothing) before.
03 Jan 2025Airtable is an impressive tool that keeps surprising me with its power. But sometimes, it seems to be missing what I assume would be very basic features.
For example, it has powerful linking capabilities; let's say you have a companies
table and an employees
table, you can have a company
field in employees
that automatically allows selecting an existing company. The mirroring effect is automatically enabled as well, when you look at your company
table, you can see all its linked employees
.
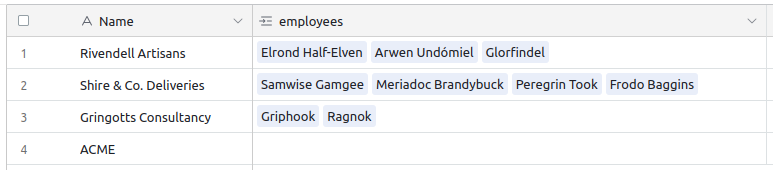
You can even use a Rollup field to, for example, gather all name
s of the linked employees. By default it's displayed as a comma-separated list of values, but in reality it returns an array-like structure, and you can call a bunch of array-related methods on it, like ARRAYUNIQUE
, ARRAYJOIN
, ARRAYCOMPACT
or ARRAYSLICE
.
But I couldn't find a way to get the length of the array. There is no ARRAYLENGTH
method.
Edit: Seems like there is a Count type that does exactly that. It was there, in plain sight, and I never saw it. It's a much better solution than the hack I'm describing here.
The workaround
Still, I found a clever / hackish way to get that information, by using a mix of string and regexp functions. Let me walk you through it:
First, I define a UUID
field in the employees
table, a formula that only contains RECORD_ID()
. That way, I have a relatively short and very unique identifier for each employee.
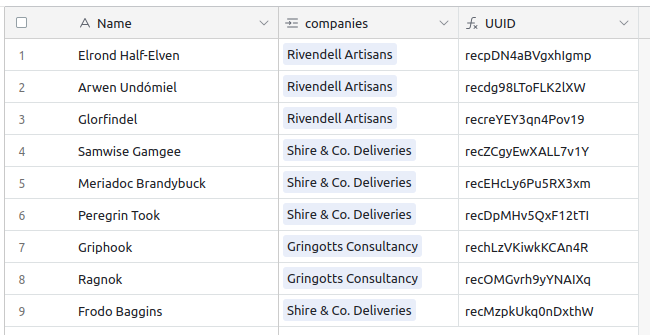
Now, let's see what we need to do in the companies
table. It is going to be a pretty long and complex formula, so we'll go step by step. You could technically put the very long formula in the Rollup, but to make it clearer, I'll create several fields, and reference them.
First, I create a an employeeCountStep1
Rollup of the UUID
field of the employees
field, but instead of joining it with the default ,
separator, I'll use a less common character, like ▮
. I could have used any character, but I find this blocky square to be more visible. So, this is our Rollup formula for now: ARRAYJOIN(ARRAYUNIQUE(values), "▮")
.
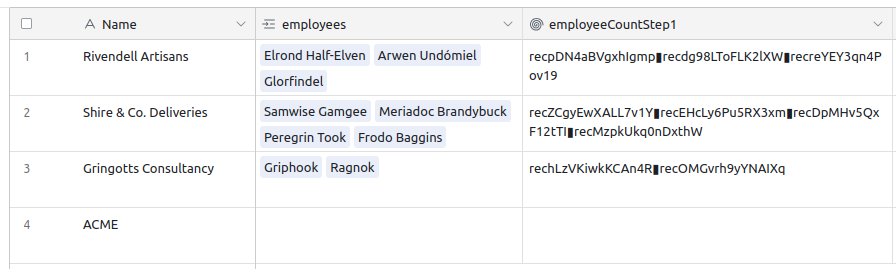
Now, as this returns a string, we'll be able to execute some regexp search and replace on it. What we'll do is remove all characters, except our blocky squares. Essentially it means replacing everything that is not ▮
, with an empty string, like this: REGEX_REPLACE(employeeCountStep1, "[^▮]", "")
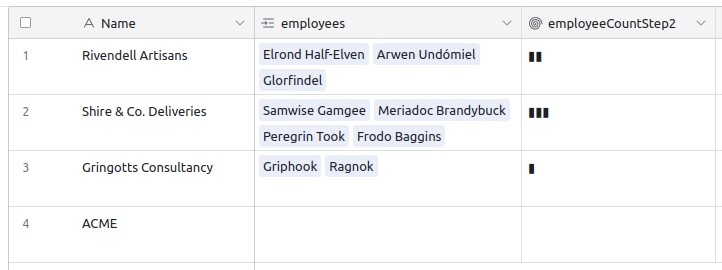
The last step is to count the number of ▮
we now have, using the LEN
method. But as the ▮
only separates values, we'll have an off-by-one error; if we have 3 employees, we'll only have two ▮
. No problem, we just need to add 1 to the total… But that means that it will also add 1 even if there are no employees… Ok, so let's wrap that in one final condition to handle that edge-case: IF(employees = "", 0, LEN(employeeCountStep2) + 1)
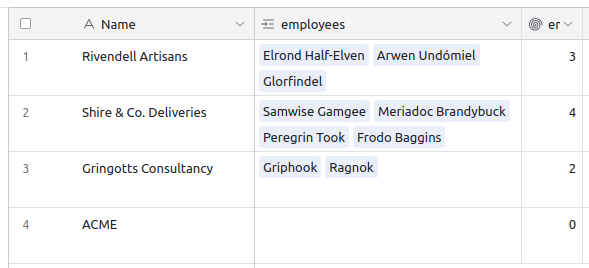
All in all, here is the final Rollup formula: IF(employees = "", 0, LEN(REGEX_REPLACE(ARRAYJOIN(ARRAYUNIQUE(values), "▮"), "[^▮]", "")) + 1)
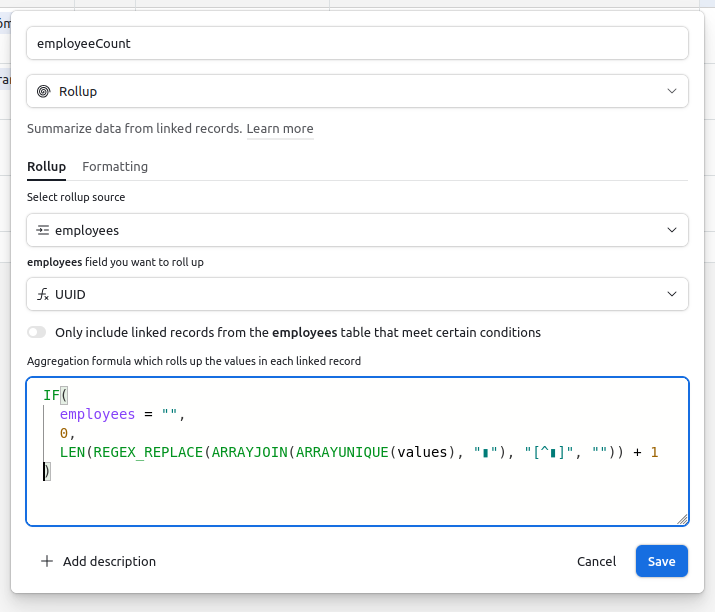
Well, that wasn't easy, but it works. It's a bit hackish, but it does the job. It's a neat trick to have in your Airtable toolkit.
02 Jan 2025When working with Airtable, I've started to develop some good practices regarding IDs in my tables.
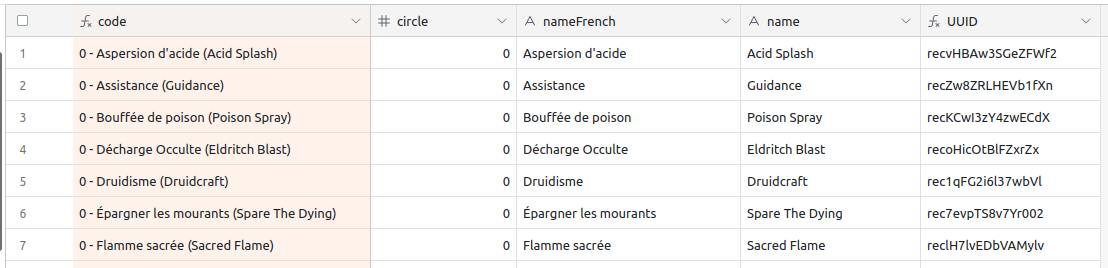
Airtable has a concept of a Primary Field, which is the "main" field of a given entry, displayed as the first column in Grid view. It can be any field of the table (even a formula aggregating several fields), and will be used in the UI to represent the row.
But Airtable also has an internal notion of a RECORD_ID()
(a string like recR5nCNhl3SHbC1z
). It's an internal ID, and if you're only using the Airtable UI, you'll never see them.
But the moment you start using the API, it's everywhere, as it's the canonical way to identify a record.
I developed the habit of making the RECORD_ID()
visible in all my tables. The first thing I do when I create a new table, is to add a field called UUID
, set it as a formula, with a value of RECORD_ID()
. That way, I have an easier mental mapping between what I can see in the UI and what I interact with in the API.
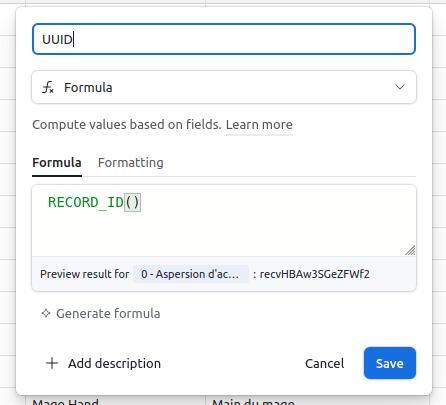
I also tend to name my Primary Field code
, and make it a concatenation of unique (but short) identifiers of my record. That way, I can easily identify them, sort them, etc. I never use that field anywhere but in the UI.
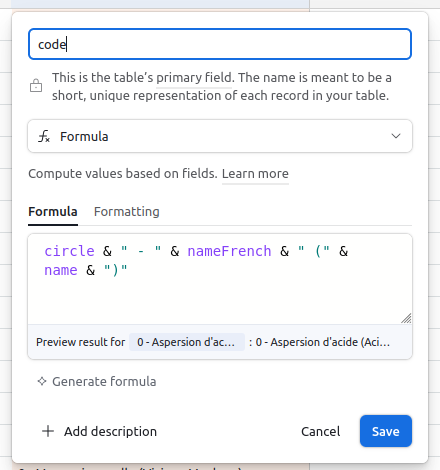
Coming from a dev background, not understanding the difference between the visible Primary Field and the hidden RECORD_ID()
has been one of the first things that tripped me up when I started plugging Airtable with Make. Having the UUID
clearly exposed in the UI and the record makes everything way simpler.